Now featuring commands executed by other players in lobby!
A ChatCommands API to use Commands over the ingame/lobby chat. I decided to write a standard-Command-API to give users the chance to do not have to use lots of different command-syntaxes from different mods.
Since this an easy-to-use API, it'll be easy to implement this api to your code.
This mod will be required soon by:
- InspirePriority
- SaveEquipment
- StealthSync (synchronization overhaul coming soon)
Pre-defined commands:
- /help [command] -> Prints some information about the given command
- /commands -> Prints all available commands
- /mods -> Prints the mods that are currently controllable by commands
- /modcommands [modname] -> Prints the available commands for the given mod
- /whoami -> Prints your own peer_id
- /whois [peer_id] -> Prints the steam name of the given peer_id. Prints "No Name" if there is no player on this peer_id or the given peer_id is yours.
For modders: If your mod uses ChatCommands and you want to be listed here, send me a PM!
How to say BLT that your mod requires ChatCommands?
-> Just add a "libraries" node into your mod.txt - file.
Example:
{
"name" : "StealthSync",
"description" : "Improves the syncronization of units in stealth mode to prevent players from going insane.",
"author" : "SerMi",
"contact" : "sermi@gpplanet.de",
"version" : "0.1",
"libraries" :
[
{
"identifier" : "ChatCommands",
"display_name" : "ChatCommands [API]"
"optional" : false
}
],
"updates" :
[
{
"revision" : 1,
"identifier" : "StealthSync"
}
],
"hooks" : [
{ "hook_id" : "lib/network/base/networkpeer", "script_path" : "lua/StealthSync.lua" },
{ "hook_id" : "lib/units/interactions/interactionext", "script_path" : "lua/StealthSync.lua" }
],
}
To use your own commands, just use the following function in your code:
ChatCommands:addCommand(commandname, modname, cmd_description, arguments, callback_function, command_type)
-> The "command_type" parameter (string) determines who has access to this command: choose between "clientonly" (default -> if nil or not given), "hostonly" (clientonly + must be host), "lobbywide" (everyone in the lobby can execute this command using the chat).
-> The "callback_function" parameter (function) is used to hold your callback-function. The API passes the entered arguments in an string-array (table) and the peer of the user who executed the command [-> function(args, user) ... end]. If your callback-function returns anything, this will be printed in the chat.
-> The "arguments" parameter (table; can even be nil) is a table holding arguments made with :
ChatCommands:newArgument(argname, value_type, required)
-> The "value_type" parameter (string) determines what values are allowed: choose between "boolean", "number", "string" and "peer_id"
-> The "required" parameter (boolean) determines if this argument is required in order to use this command or not.
Example:
ChatCommands:addCommand("thisisacmd", "mymod", "Just does something", {ChatCommands:newArgument("justanargument", "string", true), ChatCommands:newArgument("justanotherargument", "boolean", false)}, function(args, user)
... -- your callback-function
end)
For more examples see "lua/CommandUtilities.lua"
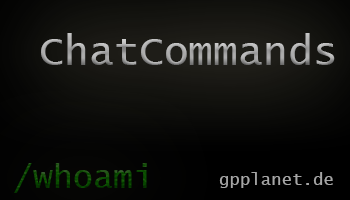
