Command Handler
Library that handles chat commands from other mods.
You're able to configure command key (commands' prefix) in the .cfg file, or via a chat command in-game. By default, it's / (forward slash). For example, you can set it to @ by typing "/set_cmd_key @" in the chat. Then all commands will start by @ instead of / (forward slash). (ie. "@ls" instead of "/ls")
Commands are also configurable, but only through the .cfg file. For example, if you find "/set_cmd_key" too long to type, you could change "set_cmd_key" to "sck" in the .cfg file, and type "/sck" instead. Other mods created by me that are using the Command Handler should also have configurable commands in their respective .cfg files. Please note that if it detects an overlapping command, they might be reverted back to their original values.
Commands
- /ls - List all available commands.
- /cls - Clear the chat window.
- /find_skill [string] - Search for a skill GUID by name
- /set_cmd_key [char] - Set a new command key
Commands are configurable in the .cfg file.
How to implement it in your mods
-
Make a reference to this .dll in your project.
-
Add the dependency in your plugin declaration.
[BepInDependency("eradev.stolenrealm.CommandHandler")] [...] public class Plugin : BaseUnityPlugin
-
Declare your commands.
In the following example, the first command would be activated by "/fc" and the second would by "/sc".The commands must be unique across all mods. If there's a conflict (due to an user changing it in their .cfg file for example), it will revert to the value declared in the
Config.Bind(...)
. If the default value is also in conflict, then it won't register.private static ConfigEntry<string> _cmdCommand1; private static ConfigEntry<string> _cmdCommand2; [...] _cmdCommand1 = Config.Bind("Commands", "command1Name", "fc", "Command 1 description"); _cmdCommand2 = Config.Bind("Commands", "command2Name", "sc", "Command 2 description");
-
Register your commands by adding this to your startup bloc (Usually
Awake()
).CommandHandler.RegisterCommandsEvt += (_, _) => { CommandHandler.TryAddCommand("PluginName", ref _cmdCommand1); CommandHandler.TryAddCommand("PluginName", ref _cmdCommand2); };
-
Register your commands' logic by adding this to your startup bloc.
CommandHandler.HandleCommandEvt += (_, command) => { if (command.Name.Equals(_cmdCommand1.Value)) { // Execute logic for command1 (/fc) } else if (command.Name.Equals(_cmdCommand2.Value)) { // Execute logic for command2 (/sc) } };
This is the bare minimum to add working chat commands to your mod. Please check the source code of my other mods that uses this library for more examples.
Bugs and suggestions
If you encounter any bugs, or have suggestions, please open an issue at https://github.com/Eradev/StolenRealmMods.
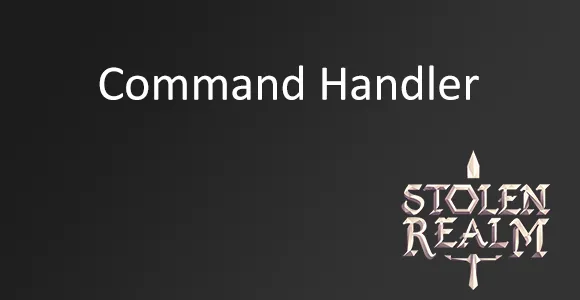