NOTE: This is only for mod developers who want to manipulate custom sounds and load soundbanks easily and that stuff, you know it
NOTE 2: This API is for easily loading soundbanks and manipulating custom sounds, it CAN'T CREATE NEW CUSTOM SOUNDS but if you want to create custom sounds then you can learn how to do it here (don't load soundbanks the way it says there, load it using this instead)
Github repository, where you can look at SynergyAPI's code and download it: https://github.com/SpecialAPI/SoundAPI
An API that allows easily manipulating and loading custom sounds.
To use this API, just
- Add it's files except files from Examples folder, SoundAPI.csproj and SoundAPI.sln, README.md, .gitignore and .gitattributes and everything from the Properties folder to your project.
- Call
SoundManager.Init()
in your module'sInit()
method. You can call it later and it shouldn't cause a lot of issues but just to be sure load it fromInit()
.
SoundAPI Guide
Various SoundAPI classes and enums
Spoiler!
CustomSwitchData
- stores information about custom switches.SoundManager
- the core class of SoundAPI that allows you to add custom switches, load soundbanks and register stop audio events.StopEventType
- an enum with all types of global stop audio events.SwitchedEvent
- stores an event name, a switch group and a switch.
Loading custom soundbanks
Spoiler!
There are four methods to load custom soundbanks - SoundManager.LoadBankFromModFolderOrZip(module, fileName)
, SoundManager.LoadBanksFromModFolderOrZip(module)
, SoundManager.LoadBankFromModProject(path)
and SoundManager.LoadBanksFromModProject()
All of them are best called in the Init() method of your module.
All of them in detail:
SoundManager.LoadBankFromModFolderOrZip(module, fileName)
loads a soundbank with the file name offileName
(fileName
doesn't need the.bnk
at the end) from your mod's folder/zip. You can use it in your module like that:this.LoadBankFromModFolderOrZip("name of your soundbank file here")
SoundManager.LoadBanksFromModFolderOrZip(module)
loads all soundbanks from your mod's folder/zip. You can use it in your module like that:this.LoadBanksFromModFolderOrZip()
SoundManager.LoadBankFromModProject(path)
loads a soundbank with the path ofpath
(again, doesn't need the.bnk
at the end) from your mod's visual studio project. The bank file NEEDS to be an Embedded Resource, OTHERWISE, IT WILL NOT LOAD. You can use it in your module like that:SoundManager.LoadBankFromModProject("path to your soundbank file in your project here")
SoundManager.LoadBanksFromModProject()
loads all soundbanks from your mod's visual studio project. Again, the bank files NEED to be Embedded Resources, OTHERWISE, THEY WILL NOT LOAD. You can use it in your module like that:SoundManager.LoadBanksFromModProject()
Registering custom stop audio events
Spoiler!
If you have a custom event that stops a certain sound/music you can register it so other "global" stop audio events stop that custom sound as well.
You can do it like this: SoundManager.RegisterStopEvent("name of your stop audio event here", (optional) stopEventTypes)
. You can add the optional stopEventTypes
if you want it to be stopped by "global" stop audio events other than the universal "Stop_SND_all"
. Example: SoundManager.RegisterStopEvent("name of your stop audio event here", StopEventType.Music (it will be stopped by "Stop_MUS_All"), StopEventType.Weapon (it will be stopped by "Stop_WPN_All"))
Adding custom switches to switch groups
Spoiler!
You can add custom switches to switch groups with SoundAPI. If you don't know what switch groups are, they are basically groups of switches. You can set a switch for a certain object and basically that will change certain sounds played by that object. There are various switch groups, like "WPN_Guns"
(used by guns to have unique sounds), "CHR_Enemy"
(used by enemies to have unique sounds). Examples of switches for "WPN_Guns"
: "Baseball"
(used by casey), "Magnum"
(used by magnum). Examples of switches for "CHR_Enemy"
: "Bullet_Bat"
(used by bullat), "Tarnisher"
(used by tarnisher).
Ok enough talking about switches and switch groups, now onto the point: you can add custom switches to switch groups by using SoundManager.AddCustomSwitchData(switchGroup, switchValue, originalEventName, replacementEvents)
What the arguments mean:
switchGroup
- the switch group to add the switch too.switchValue
- the name of the custom switch.originalEventName
- the name of the original event that will be replaced.replacementEvents
- events that will be played in place of the original event. They can be eitherstring
(example:"Play_WPN_kthulu_blast_01"
) orSwitchedEvent
(example:new SwitchedEvent("Play_WPN_Gun_Shot_01" (the event's name), "WPN_Guns" (the switch group), "jk47" (the switch that will temporarily be turned on to play the event))
). You don't have to make a new array to add the replacement events, you can include them as individual arguments and they will all be recognised and grouped. You don't have to worry about making them all the same type (allstring
or allSwitchedEvent
), you can mix the two types.
Examples
Spoiler!
There's a file in the Examples folder called ExampleModule.cs
. It shows how to use various SoundAPI methods and their arguments. You shouldn't add it to your project, but you can look at it when you need an example of how to use a method or it's argument.
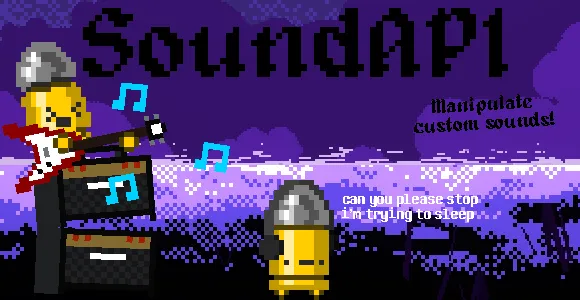
