NOTE: This is only for mod developers who want to add custom synergies blah blah blah
Github repository, where you can look at SynergyAPI's code and download it: https://github.com/SpecialAPI/SynergyAPI
An API that allows well... you guessed it, create custom synergies.
To use this API, just
- Add it's files except files from Examples folder, SynergyAPI.csproj and SynergyAPI.sln, README.md, .gitignore and .gitattributes and everything from the Properties folder to your project.
- Call SynergyBuilder.In- wait, you don't even need to do that! SynergyAPI is smart and inits itself for you when you want to create a synergy!
SynergyAPI Guide
Various SynergyAPI classes
Spoiler!
AdvancedStringDB
andAdvancedStringDBTable
- they exist. I guess you can set synergy strings and complex strings with them? But I don't see any reason for you to do that.SynergyBuilder
- it builds synergies! What a surprise! Who would have ever expected that?AdvancedCompanionSynergyProcessor
,AdvancedDualWieldSynergyProcessor
,AdvancedGunFormeSynergyProcessor
,AdvancedGunFormeData
,AdvancedHoveringGunSynergyProcessor
,AdvancedInfiniteAmmoSynergyProcessor
,AdvancedTransformGunSynergyProcessor
- components and other stuff for dual wield, gun transform, companion, gun forme, hovering gun and infinite ammo synergies.
Adding custom synergies
Spoiler!
To add a custom synergy use SynergyBuilder.CreateSynergy(name, mandatoryIds OR mandatoryItems, optionalIds OR optionalItems, activeWhenGunsUnequipped, statModifiers, ignoreLichsEyeBullets, numberObjectsRequired, suppressVfx, requiresAtLeastOneGunAndOneItem, bonusSynergies)
What the arguments mean:
name
- if it isn't obvious already, the name of the synergy.mandatoryIds
- either the numerical ids or the MtG console ids of the items that are all required for the synergy completion ORmandatoryItems
- items required for the synergy completion. The synergy will only be completed if the player has ALL of the required items.optionalIds
- either the numerical ids or the MtG console ids of the "filler items" (items that fill empty spaces in the synergy that were not filled with mandatory items. you can get any of those to fill the spaces) ORoptionalItems
- the "filler items" (I already explained what that is before) for the synergy. You're not required to use this argument, it defaults to nothing.activeWhenGunsUnequipped
- if false, the synergy and it's stat boosts (more about that later) will only be active when you're holding any gun from the synergy list. You're not required to use this argument, it defaults to true.statModifiers
- stat modifiers the player will get when the synergy is active. You're not required to use this argument, it defaults to nothing.ignoreLichsEyeBullets
- if true, the player will not be able to complete the synergy with lich's eye bullets. I'm not sure why you would want that but I included it anyways. You're not required to use this argument, it defaults to false.numberObjectsRequired
- number of items the player needs to get from the synergy item list for the synergy to be active. You're not required to use this argument, it defaults to 2.suppressVfx
- if true, the synergy will not create the usual "synergy vfx" and also the item get notification will not show the synergy. Again, I'm not sure why would anyone want that but I still added it. You're not required to use this argument, it defaults to false.requiresAtLeastOneGunAndOneItem
- if true, the synergy will only activate if the player has at least one gun and one item from the synergy item list. You're not required to use this argument, it defaults to false.bonusSynergies
- list of "Bonus Synergies" that the player will get when the synergy activates. Bonus Synergies are how base game synergies are detected. IT DOES NOT WORK FOR MODDED SYNERGIES so I'm not sure how it will be useful but I still added it. You're not required to use this argument, it defaults to nothing.
Checking if a player has a synergy
Spoiler!
To check if player has a custom synergy use playerThatYouWantToCheck.PlayerHasActiveSynergy(theNameOfTheSynergyYouWantToCheck)
. THIS ONLY WORKS ON CUSTOM SYNERGIES FROM YOUR MOD THIS DOES NOT WORK FOR CUSTOM SYNERGIES FROM OTHER MODS AND ALSO THIS DOES NOT WORK FOR BASEGAME SYNERGIES. To check if player has a basegame synergy use playerThatYouWantToCheck.HasActiveBonusSynergy(theBonusSynergyTypeForThatSynergy)
.
If you want to check if any player has a custom synergy use SynergyBuilder.AnyoneHasActiveSynergy(theNameOfTheSynergyYouWantToCheck)
. AGAIN, THIS ONLY WORKS ON CUSTOM SYNERGIES FROM YOUR MOD THIS DOES NOT WORK FOR CUSTOM SYNERGIES FROM OTHER MODS AND ALSO THIS DOES NOT WORK FOR BASEGAME SYNERGIES. If you want to check if any player has a basegame synergy use PlayerController.AnyoneHasActiveBonusSynergy(theBonusSynergyTypeForThatSynergy, out _)
.
Adding dual wield, hovering gun, gun transformation, companion, infinite ammo and gun forme synergies
Spoiler!
Adding a dual wield synergy between two guns: SynergyBuilder.AddDualWieldSynergyProcessor(firstgun, secondgun, synergyname)
Adding a infinite ammo synergy to a gun gun.AddInfiniteAmmoSynergyProcessor(synergyname, addsInstantReload)
Adding a companion synergy to a gun, passive or active item: itemOrGun.AddCompanionSynergyProcessor(synergyname, doesntRequireSynergy, activeWhenNotHeld (only for guns), companionGuid)
Adding a gun forme synergy base to a gun: gun.AddGunFormeSynergyProcessor()
NOTE: THIS METHOD DOES NOT ACTUALLY ADD ANY FORMES, IT JUST ADDS THE BASE SO YOU CAN ADD AS MANY FORMES AS YOU CAN LATER, SO YOU NEED TO SAVE THE THING IT RETURNS
Adding a new forme to a gun forme base: formeBase.AddForme(requiresSynergy, synergyname, formeGunId)
Adding a transform gun synergy to a gun: gun.AddTransformGunSynergyProcessor(synergyname, synergyFormId, shouldResetAmmoAfterTransformation, resetAmmoCount)
Adding a hovering gun synergy to a passive item or a gun: itemOrGun.AddHoveringGunSynergyProcessor(synergyname, hoveringGunId, usesMultipleIds, multipleIds, positionType, aimType, fireType, fireCooldown, fireDuration, firesOnlyOnEmptyReload, shootSoundEffect, onEveryShotSoundEffect, onFinishedShootingSoundEffect, spawnCondition, numToSpawn, damageTriggerDuration, consumesOriginalGunAmmo, chanceToConsumeOriginalGunAmmo)
NOTE: YOU WILL NEED TO ADD SYNERGIES WITH THE SAME NAMES AS synergyname
FROM THEIR RESPECTIVE METHODS YOURSELF, THESE METHOD DO NOT AUTOMATICALLY BUILD SYNERGIES.
Adding new items to existing synergies
Spoiler!
To add a new item to an existing synergy you need to do either item.AddItemToSynergy(synergyType OR synergyNameKey)
or SynergyBuilder.AddItemToSynergy(synergyType OR synergyNameKey, itemId)
Examples
Spoiler!
There's a file in the Examples folder called ExampleModule.cs
. It shows how to use various SynergyAPI methods and their arguments. You shouldn't add it to your project, but you can look at it when you need an example of how to use a method or it's argument.
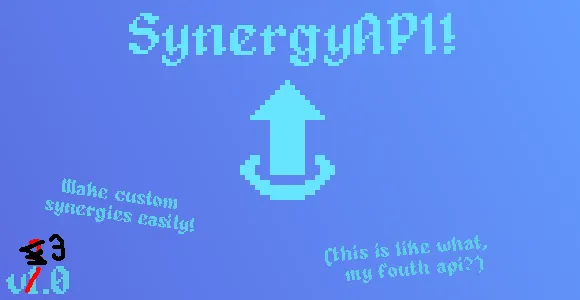
