NOTE: This is only for mod developers who want to add custom challenges to challenge mode.
Special thanks to Some Bunny for making the Chamber's Curse example challenge!
Github repository, where you can look at ChallengeAPI's code and download it: https://github.com/SpecialAPI/ChallengeAPI
An API that allows easily adding new challenge mode challenges.
To use this API, just
- Add it's files except files from Examples folder, ChallengeAPI.csproj and ChallengeAPI.sln, README.md, .gitignore and .gitattributes and everything from the Properties folder to your project.
- Call
ChallengeBuilder.Init()
in yourETGModule.Start()
method.
ChallengeAPI Guide
Various ChallengeAPI classes and enums
Spoiler!
ChallengeBuilder
- the core class that can build challenges.ChallengeTools
- a class containing all tools used by ChallengeAPI.dfLanguageExtraStringHolder
- it just exists.FakePrefabHandler
- allows you to mark objects as fake prefabs.ResourceGetter
- allows you to get resources from your project.
Adding a custom challenge
Spoiler!
To add a custom new challenge, you will need to use this method: ChallengeBuilder.BuildChallenge
<T
>([Optional] GameObject go, string challengeIconPath OR Texture2D challengeIcon, string challengeName, [Optional] bool validInBossRooms, [Optional] List
<ChallengeModifier
> mutuallyExclusive, [Optional] GlobalDungeonData.ValidTilesets excludedTilesets, [Optional] Dictionary
<GlobalDungeonData.ValidTilesets, int
> floorsWithCustomSlotRequirement, [Optional] bool addToNormalChallengeManager, [Optional] bool addToMegaChallengeManager)
What do the arguments mean:
[Optional] GameObject go
- the Game Object that will become the base for the challenge modifier.string challengeIconPath
- the filepath to the challenge icon in your project ORTexture2D challengeIcon
- the texture that will be used as the challenge's icon.string challengeName
- the challenge name that will be shown during challenge modifier announcement.[Optional] bool validInBossRooms
- should the challenge should appear in boss rooms or not. If not given, defaults tofalse
, making the challenge able to appear in boss rooms.[Optional] List
<ChallengeModifier
>mutuallyExclusive
- list of challenges your challenge modifier won't be able to get paired with.[Optional] GlobalDungeonData.ValidTilesets excludedTilesets
- a floor that the challenge won't be able to appear on. Ifnull
or not given, the challenge will be able to appear on all floors.[Optional] Dictionary
<GlobalDungeonData.ValidTilesets, int
>floorsWithCustomSlotRequirement
- a dictionary in which the key is the floor and the value is the amount of slots this challenge modifier uses on that floor. If null or not given, the challenge will use the default amount of slots on all floors. (the default amount of slots is 1)[Optional] bool addToNormalChallengeManager
- should the challenge be added to normal challenge mode challenge pool or not.[Optional] bool addToMegaChallengeManager
- should the challenge be added to double challenge mode challenge pool or not.
When using ChallengeBuilder.BuildChallenge
<T
>()
, the T
in <> needs to be replaced with your challenge modifier's class name.
Using ChallengeBuilder.BuildChallenge
<T
>()
will return a ChallengeDataEntry
. If you want to edit your challenge modifier after it's creation, you can access it like this: ReturnedChallengeDataEntry.challenge
.
Adding a custom boss challenge
Spoiler!
NOTE: You CAN'T add custom challenges to minibosses. ONLY to bosses.
To add a boss challenge, you will need to use this method: ChallengeBuilder.BuildBossChallenge(string name, List<AIActor> bosses OR List<string> bossGuids, int numChallengesToUse, List<ChallengeDataEntry> challenges OR List<ChallengeModifier> modifers, [Optional] bool addToNormalChallengeManager, [Optional] bool addToMegaChallengeManager)
What do the arguments mean:
string name
- the name of the boss challenge. While it's not seen anywhere in the game, trust me, it's required.List<AIActor> bosses
- List of bosses to which the challenge will be attached to ORList<string> bossGuids
- Guids of bosses to which the challenge will be attached to.int numChallengesToUse
- How many random challenges the game will pick from thechallenges
/modifers
list.List<ChallengeDataEntry> challenges
- list of challenge datas the challenge modifiers of which will be used in the boss challenge ORList<ChallengeModifier>
- list of challenge modifiers that will be used in the boss challenge.[Optional] bool addToNormalChallengeManager
- should the boss challenge be added to normal challenge mode's boss challenge list or not.[Optional] bool addToMegaChallengeManager
- should the boss challenge be added to double challenge mode's boss challenge list or not.
You can get the bosses for the bosses
argument using Game.Items[enemyConsoleId]
.
You can find a list of all enemy guids here: https://github.com/ModTheGungeon/ETGMod/blob/master/Assembly-CSharp.Base.mm/Content/gungeon_id_map/enemies.txt
And lastly you can get base-game challenge modifiers using ChallengeBuilder.ChallengeManagerPrefab.FindChallenge<ChallengesClassName>()
Examples
Spoiler!
There is an Examples folder in ChallengeAPI. You don't need to add it to your project, but you can look at the examples when you need an example of something in ChallengeAPI.
The examples:
ExampleModule
- an example module that shows how to setup ChallengeAPI, build a challenge, etc.ExampleChallengeModifier
- an example challenge modifier that prints messages to console when it starts or ends.ChambersCurseChallenge
- a more complex example challenge modifier that spawns dragun boulders at the player's position from time to time. It's made by Some Bunny by the way.ExampleBossChallenge
- an examle boss challenge modifier that multiplies the maximum health of all bosses by it'shealthMultiplier
variable.
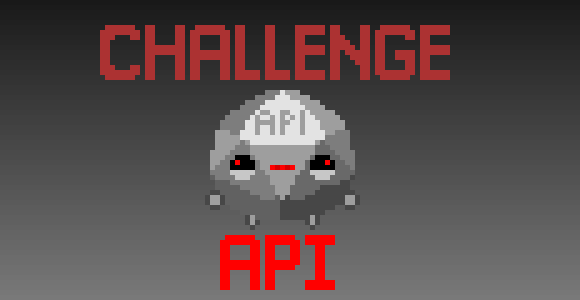
