This mod will allow you to set a custom level cap. When a monster at or above that level would level up from gaining exp after a battle, they'll instead reset their exp to 0 and not gain a level. This mod is intended for use in low level runs so you can set your own custom level cap and prevent your monsters from leveling up beyond that. It doesn't offer a way to lower your monsters' levels (hopefully yet).
Instructions on how to use the mod are in the "ReadMe" text file which comes with it.
The custom level cap can be set in a config file in BepInEx's config file. It should be generated when you first launch the game. You'll want to close the game after that in order to set the level cap in the config file.
idk how this mod will work if you have a monster already above the custom level cap and then use a level badge. It should work find or just reset their exp. Nothing game breaking.
Sourcecode:
using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
using BepInEx;
using BepInEx.Configuration;
using MonoMod;
using UnityEngine;
namespace CustomLevelCap
{
[BepInDependency("evaisa.MonSancAPI")]
[BepInPlugin("PopePius.CustomLevelCap", "CustomLevelCap", "1.0.0")]
public class Class1 : BaseUnityPlugin
{
public int customLevelCap = 40;//determines the max level the player's monsters can be leveled up to
public static ConfigEntry<int> levelCapConfig { get; set; }//reference to a config file containing the custom level cap
public void Awake()
{
Logger.LogMessage("Loaded Custom Level Cap");
levelCapConfig = Config.Bind<int>(
"Custom Level Cap Config",
"Custom Level Cap",
40,
"This value determines the maximum level your monsters can level up to. " +
"After a battle, if a monster levels up from battle and is at or above this value," +
"They'll instead stay at the same level and their exp will reset back to 0.");//builds the config file with 40 as teh default value
customLevelCap = levelCapConfig.Value;
On.Monster.LevelUp += (orig, self) =>
{
//if the monster's level is greater than or equal to the custom level cap and isn't
//equal to the normal level cap
if (self.Level >= customLevelCap && self.Level != GameController.LevelCap)
{
self.CurrentExp = 0;
self.ExpNeeded = Monster.CalculateNeededExperience(self.Level);
return;
//resets the exp and returns out in order to no execute the normal function
}
else
{
orig(self);//runs the normal function
}
};
}
}
}
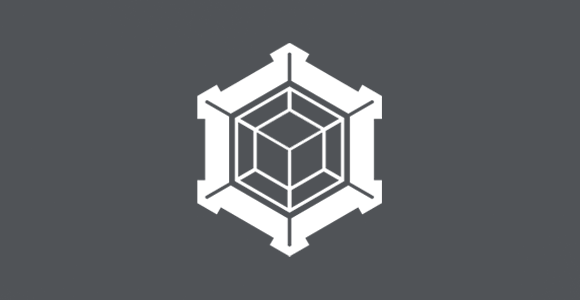
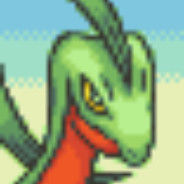